C# Tip Article
Entity Framework 6 SQL Server Connection String
1. Entity Framework 6 (EF6) uses LocalDB by default.
When EF6 is installed (typically from Nuget), the following default settings are added to Web.config (for web application) or App.config (for non-web application). As you can see defaultConnectionFactory is set to LocalDbConnectionFactory.
<entityFramework> <defaultConnectionFactory type="System.Data.Entity.Infrastructure.LocalDbConnectionFactory, EntityFramework"> <parameters> <parameter value="mssqllocaldb" /> </parameters> </defaultConnectionFactory> </entityFramework>
If you run the project for the first time, new database will be created under "(LocalDB)\MSSQLLocalDB" (in case of SQL 2014 LocalDB) and DB name will be the concatenation of namespace and DbContext class name. Please note that localdb name can vary. If you have SQL 2012, it is under (LocalDB)\v11.0, so you need to specify <parameter value="mssqllocaldb" /> instead.
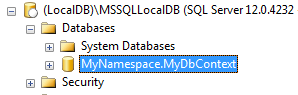
2. How to Use SQL Server instead of LocaDB in EF6
In order to use SQL Server instance instead of LocaDB, change defaultConnectionFactory to SqlConnectionFactory and set DB connection string to its parameter as below.
<defaultConnectionFactory type="System.Data.Entity.Infrastructure.SqlConnectionFactory, EntityFramework"> <parameters> <parameter value="Data Source=.;Integrated Security=True;MultipleActiveResultSets=True" /> </parameters> </defaultConnectionFactory>
As for LocaDB, if you run the project for the first time, new database will be created under local SQL server (as specified in [Data Source] param) and DB name will be the concatenation of namespace and DbContext class name.
People may not want to use auto-generated DB name in SQL Server. Unfortunately, we cannot specify database name in defaultConnectionFactory setting. But, you can specify DB name in DbContext class. Below example specifies MyDB in base(), and so it will create MyDB database instead of MyNamespace.MyDbContext.
namespace MyNamespace { public class MyDbContext : DbContext { public MyDbContext() : base("MyDB") { } //... } }