C# Tip Article
Using TLS 1.2 in C#
.NET 4.0 (or below) supports SSL 3.0 and TLS 1.0 (a successor of SSL 3.0). SSL and TLS are often used when we use https protocol. To simply check out the real example, we can write a small example using WebRequest. Below code snippet calls google.com with https. Let's assume that it is compiled with .NET 4.0.
string url = "https://www.google.com"; var req = (HttpWebRequest)WebRequest.Create(url); req.Method = "GET"; var resp = req.GetResponse(); var outStream = resp.GetResponseStream(); string output = ""; using (StreamReader rdr = new StreamReader(outStream)) { output = rdr.ReadToEnd(); } Debug.WriteLine(output);
To see https communication, we can use Fiddler. When checking web response in Fiddler, we see TLS 1.0 is used.
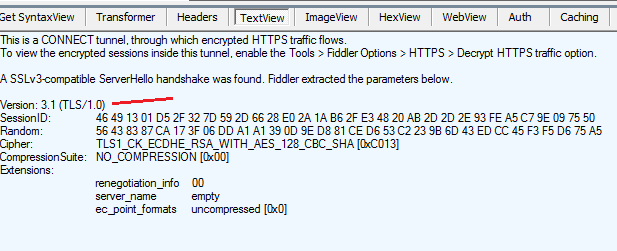
The problem is SSL3 and TLS1.0 are vulnerable to POODLE attack, one of man in the middle (MITM) security attack. So if you have .NET 4.0 applications which handle security sensitive data such as credit card processing, you might want to migrate to .NET 4.5 or above.
.NET 4.5 or above supports TLS 1.1 and TLS 1.2 that does not have POODLE vulnerability. One caveat is simply upgrade to .NET 4.5 does not automatically solve the problem. That is because the default protocol is TLS 1.0 in .NET. So in order to use TLS 1.2 or TLS 1.1 in .NET 4.5+, you will need to set SecurityProtocol as below before any WebRequest calls.
System.Net.ServicePointManager.SecurityProtocol = System.Net.SecurityProtocolType.Tls11 | System.Net.SecurityProtocolType.Tls12; var req = (HttpWebRequest)WebRequest.Create(url); req.Method = "GET"; ...
Now you will see TLS 1.2 in Fiddler.
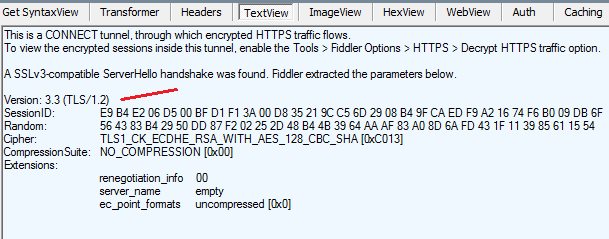
NOTE: It is OK to include TLS 1.0 along with TLS1.1 and TLS1.2 in many cases, since the client will negotiate with server and take the highest protocol that server provides. As long as server provides TLS 1.1 or TLS 1.2, the client will use theTLS 1.1 or 1.2.