C# Tip Article
How to change system time in C#?
In C#, DateTime struct does not have any method to set system time. How can we change system time in C#?
We need to use Win32 API via P/Invoke. SetSystemTime() API sets new system time, and GetSystemTime() returns current system time. Both SetSystemTime() and GetSystemTime() are using UTC time. To get UTC system time, you can also use DateTime.UtcNow.
Here is the code example that changes system time. It sets Utc datetime in SYSTEMTIME and call SetSystemTime() API.
using System; using System.Runtime.InteropServices; namespace Time { class Program { static void Main(string[] args) { // Change System Time // SetSystemTime expects the SYSTEMTIME in UTC // (ex) 2015-8-12 11:30:15 UTC DateTime date = new DateTime(2015, 8, 12, 11, 30, 15, 0, DateTimeKind.Utc); SYSTEMTIME systime = new SYSTEMTIME(date); SetSystemTime(ref systime); // Get UTC time Console.WriteLine("{0:yyyy-MM-dd hh:mm:ss}", DateTime.UtcNow); } [DllImport("kernel32.dll")] static extern bool SetSystemTime(ref SYSTEMTIME time); [StructLayout(LayoutKind.Sequential)] public struct SYSTEMTIME { public ushort Year; public ushort Month; public ushort DayOfWeek; public ushort Day; public ushort Hour; public ushort Minute; public ushort Second; public ushort Milliseconds; public SYSTEMTIME(DateTime dt) { Year = (ushort)dt.Year; Month = (ushort)dt.Month; DayOfWeek = (ushort)dt.DayOfWeek; Day = (ushort)dt.Day; Hour = (ushort)dt.Hour; Minute = (ushort)dt.Minute; Second = (ushort)dt.Second; Milliseconds = (ushort)dt.Millisecond; } } } }
One caveat about changing system time is that the system time can be reset by Windows Time Service (especially if the the PC is joined in a domain). To check to see if the machine is using Windows Time service, run this command with admin permission. And if you want to resync time with time server, use /resync option.
REM query time server C> w32tm /query /source REM resync time C> w32tm /resync
Another caveat is that if the time is out of range, your internet browser might throw an error.
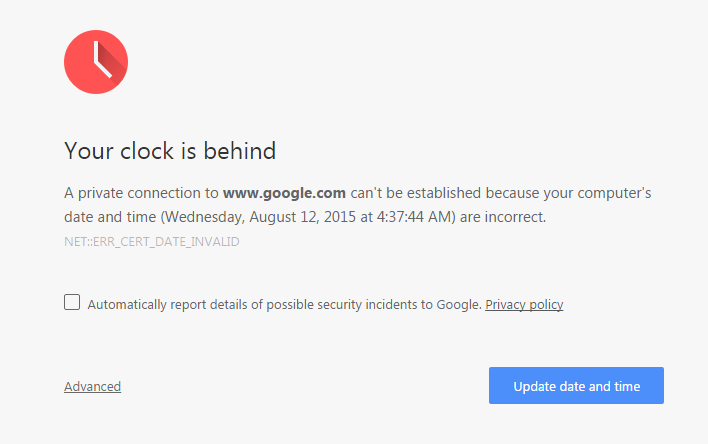