C# Tip Article
Ambiguous method overloading - string vs string[] args
A question from a Facebook C# group.
How can we get around the ambiguous method overloading error in the C# code snippet below when null is passed?
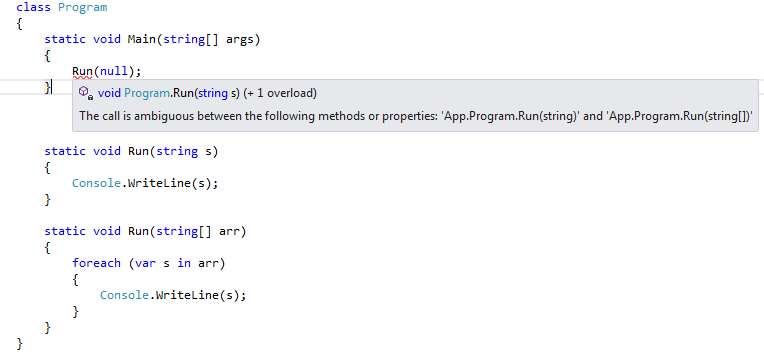
A questioner said he added new Run(string) method on top of old Run(string[]) method.
One way of avoiding 'ambiguous method overloading error' is simply casting the input argument when one calls it.
For example, to call Run(string[]) method, one can say:
Run((string[]) null);
Another way is rethink why we need to add Run(string) in the first place.
Are those methods basically the same methods, other than single string vs. multiple strings input?
If so, is it possible not to add new method and change Run(string[]) to Run(params string[])?
In C#, params keyword allows 3 things - (1) variable parameters, (2) array of the parameter type, and (3) null.
So one string can be accepted (as a variable parameter), multiple strings are accepted (array) and also null can be passed on the method. So one can reconsider if the following approach is appropriate for one's need.
static void Run(params string[] arr) { foreach (var s in arr) { Console.WriteLine(s); } }